It is given that the sales of ice cream is three times as shakes.
Let x be the number of ice creams and y be the number of shakes,
So x=3y.
Also the total sales if ice cream is $2 and shake is $9 is $9600 so it follows:
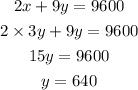
Since x=3y so it follows that x=1920.
So Number of shakes sold is 640 and number of ice cream cones sold is 1920.